How to check for an active Internet Connection on iPad (iPhone) SDK
Sometimes, you need to find out whether your application is connected to the Internet. Is again a few possibilities, but not all are correct.
Test open web page
Simple solution is to try visit a website. Where you can download the content, we have connection to internet.
1 2 3 |
+ (BOOL) connectedToNetwork{ return ([NSString stringWithContentsOfURL:[NSURL URLWithString:@"http://google.com"]] != NULL ) ? YES : NO; } |
This method is not bad, but unfortunately the function stringWithContentsOfURL is deprecated.
Use Reachability class
Second solution is use “Reachability” class from apple (http://goo.gl/kSHn3) and implement internet checking with this.
In this case we’ll download Reachability.m and Reachability.h and add this class into our project.
Now we can create a wrapper for example, which will examine information about the connection
File Internet.h
1 2 3 4 5 6 7 |
@interface Internet : NSObject + (BOOL) connectedToNetwork; + (BOOL) connectedToWifi; + (BOOL) connectedToWWAN; @end |
File Internet.m
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 |
#import "Internet.h" #import "Reachability.h" @implementation Internet + (NetworkStatus)getNetworkStatus{ Reachability *reachability = [Reachability reachabilityForInternetConnection]; NetworkStatus networkStatus = [reachability currentReachabilityStatus]; return networkStatus; } + (BOOL) connectedToNetwork{ return !([self getNetworkStatus] == NotReachable); } + (BOOL) connectedToWifi{ return !([self getNetworkStatus] == ReachableViaWiFi); } + (BOOL) connectedToWWAN{ return !([self getNetworkStatus] == ReachableViaWWAN); } @end |
Now we have three static functions which return information about internet connection.
Possible problems
It is possible that your application does not compile, and you get an error:
1 2 3 4 5 6 7 8 9 10 |
Cast of C pointer type 'void *' to Objective-C pointer type 'NSObject *' requires a bridged cast 'NSAutoreleasePool' is unavailable: not available in automatic reference counting mode 'NSAutoreleasePool' is unavailable: not available in automatic reference counting mode Cast of C pointer type 'void *' to Objective-C pointer type 'Reachability *' requires a bridged cast 'release' is unavailable: not available in automatic reference counting mode ARC forbids explicit message send of 'release' Implicit conversion of Objective-C pointer type 'Reachability *' to C pointer type 'void *' requires a bridged cast ARC forbids explicit message send of 'dealloc' ARC forbids explicit message send of 'autorelease' ARC forbids explicit message send of 'autorelease' |
This fix quite easily, disabling automatic reference in the project:
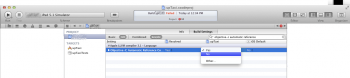
Last error which you can see is:
1 |
Declaration of 'struct sockaddr_in' will not be visible outside of this function |
Repair is again simple, just add into the header file Reachability.h this line:
1 |
#import <netinet/in.h> |