iOs 6: How to open the native application with navigation
Ios version 6 and higher deprecated Google Maps. How to properly open and navigate user to the target object in a native application?
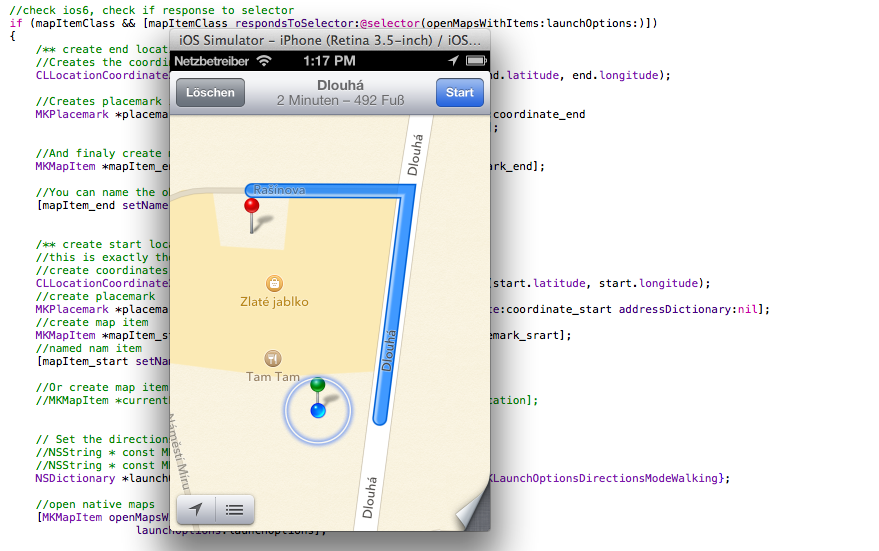
We can use MKMapItem class, but this class was publicly available starting with iOS 6.0.
In this case you typically check whether that class is nil. Unfortunately, this test is not correct accurate for MKMapItem. Although this class was available with iOS 6.0, it was in development prior to that. But Apple does not recommend using it in earlier versions
Correct test might loog like the following:
1 2 3 4 |
Class itemClass = [MKMapItem class]; if (itemClass && [itemClass respondsToSelector:@selector(openMapsWithItems:launchOptions:)]) { // Use class } |
We can test weather the class object is not nill and openMapsWithItems:launchOptions: class method exists.
If everything is ok, you can use this class, otherwise open Google Maps in browser.
How to use MKMapItem
As you can see in the following code, using is simple.
We create start and end coordinates and open maps with navigation.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 |
- (void)navigateToStreetAction { //location is valid -> navigate CLLocationCoordinate2D start = self.locationManager.location.coordinate; CLLocationCoordinate2D end = CLLocationCoordinate2DMake([Street.latitude doubleValue], [Street.longitude doubleValue]); //create class object Class mapItemClass = [MKMapItem class]; //check ios6, check if response to selector if (mapItemClass && [mapItemClass respondsToSelector:@selector(openMapsWithItems:launchOptions:)]) { /** create end location **/ //Creates the coordinates for navigation CLLocationCoordinate2D coordinate_end = CLLocationCoordinate2DMake(end.latitude, end.longitude); //Creates placemark into map MKPlacemark *placemark_end = [[MKPlacemark alloc] initWithCoordinate:coordinate_end addressDictionary:nil]; //And finaly create map Item into map MKMapItem *mapItem_end = [[MKMapItem alloc] initWithPlacemark:placemark_end]; //You can name the object [mapItem_end setName:business.name]; /** create start location **/ //this is exactly the same as the previous case //create coordinates CLLocationCoordinate2D coordinate_start = CLLocationCoordinate2DMake(start.latitude, start.longitude); //create placemark MKPlacemark *placemark_srart = [[MKPlacemark alloc] initWithCoordinate:coordinate_start addressDictionary:nil]; //create map item MKMapItem *mapItem_start = [[MKMapItem alloc] initWithPlacemark:placemark_srart]; //named nam item [mapItem_start setName:@"Start"]; //Or create map item with current location //MKMapItem *currentLocationMapItem = [MKMapItem mapItemForCurrentLocation]; // Set the directions mode //NSString * const MKLaunchOptionsDirectionsModeDriving; //NSString * const MKLaunchOptionsDirectionsModeWalking; NSDictionary *launchOptions = @{MKLaunchOptionsDirectionsModeKey : MKLaunchOptionsDirectionsModeWalking}; //open native maps [MKMapItem openMapsWithItems:@[mapItem_start, mapItem_end] launchOptions:launchOptions]; //or navigate from current location // [MKMapItem openMapsWithItems:@[currentLocationMapItem, mapItem_end] launchOptions:launchOptions]; }else{ //create url with start and end coordinates NSURL *url = [[[NSURL alloc] initWithString: [NSString stringWithFormat:@"http://maps.google.com/maps?saddr=%1.6f,%1.6f&daddr=%1.6f,%1.6f", start.latitude, start.longitude, end.latitude, end.longitude]] autorelease]; //open google maps in safari [[UIApplication sharedApplication] openURL:url]; } } |
Better to test as first if Google maps app is available, default Apple maps sucks.
Google Maps in the browser are always available. This is a demonstration when we need use native maps.
Btw why do you create CLLocationCoordinate2D coordinate_end and CLLocationCoordinate2D coordinate_start when you already have CLLocationCoordinate2D end and CLLocationCoordinate2D start?
Yes, you’re right, you do not have to do a new instance, I wrote this more for better readability in code.
Hey Hi,
When i tried with the above i am getting error like “A route to the nearest road can not be determined “. Could you please explain me why
/?